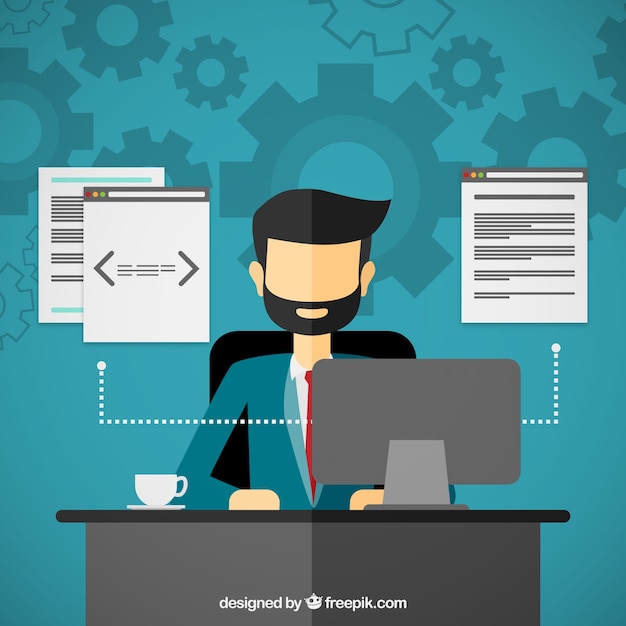
Topics to Study When Learning Java:
1.) Vocabulary:
I have said this in previous posts and I will continue saying it until the day my hands fall off and I am not able to type anymore, vocabulary is key in the language of Java. Since you cannot write code in Java like we speak, then you must learn the Java vocabulary to put in your code so that the computer can read and understand it. Let's say that you want to write code that says if number 'a' is larger than number 'b', then print out "yes". If not, then you want it to print out no. This code would look something like this:
int a = 5;
int b = 4;
if (a > b)
{
System.out.println("yes");
}
else
{
System.out.println("no");
}
If you did not know the words to put in the code, the program will not compile, causing you, the developer, to have a lot of headaches. So, save yourself some headaches by just learning the vocabulary. It will quickly become second nature to know what they mean and what they do.
2.) Loops:
Loops are a very helpful method to use in your programming when want to repeat a process until you get a result. One of these loops is referred to as a 'While Loop' and is usually used to cause a process to be ran a specific number of times. Though it seems relatively simple, these little guys can be a pain to trace through and to implement at higher level programming. Also, they are so widely used, you can't really just skip over the topic and expect to do good in in your assignments. If you just read up on them a little, you will lean that they can make your program 100 lines shorter and a lot easier.
3.) Arrays:
Imagine a list that can hold anything from numbers to strings to boolean equations. That is basically what an array is in Java. An array is just a list that you can store values in, separating them into cells of the array. Here is a short visual:
[ a1, a2, a3, a4 ] //This is your starting list array. 'a1', 'a2', etc. are symbols of the numbers
a1 = 5; //These are declare your symbols to a certain number
a2 = 4;
a3 = 1;
a4 = 8;
[ 5, 4, 1, 8 ] //This is your array list with the number values in them
This concept can be harder to follow when you get further in depth with what you can do with arrays. Once you learn how to use these effectively, you can make your code a lot shorter and you can do a lot more with your programming.
4.) Linked Lists:
Linked Lists are similar to arrays, but they hold addresses rather than just values. This concept has a lot to do with networks and connections between nodes. A node is one individual address/value. You can connect these nodes and tell them where to the next node to go to is, making a larger network of nodes fully connected. This concept can be an extremely hard concept to grasp as it can do so much within your code and following through its path can be a pain in the rear. That is why this is so crucial to learn properly and understand it thoroughly.
5.) Method Creation:
A method is a set of code that is written for a given individual purpose to assist the entire program. An example of this would be if you were making a calculator program, you would make a method for the add function, the subtract function, etc. You can have hundreds of methods in one program, so it is essential that you get the setup correct to save yourself some headache. This isn't a harder topic to understand, but it is still heavily relied on for programming, making it at the bottom of this list.
I suggest getting as much knowledge of Java as you can in all topics, not just these listed. This list is to just give you a stepping stone that will lead to more amazing topics of the language.
-Stay Geeky!
No comments:
Post a Comment